Related Topics
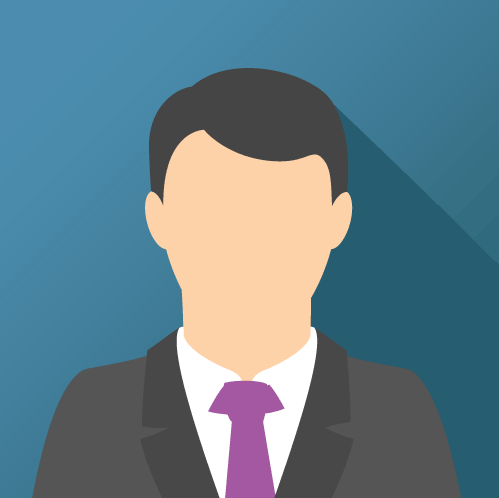
Kumar
Make predictions using dataset in Keras.
Python code for making a regression prediction in Keras:
from keras.models import Sequential
from keras.layers import Dense
from sklearn.datasets import make_regression
from sklearn.preprocessing import MinMaxScaler
from numpy import array
X, y = make_regression(n_samples=1000, n_features=2, noise=0.2, random_state=1)
scalarX, scalarY = MinMaxScaler(), MinMaxScaler()
scalarX.fit(X)
scalarY.fit(y.reshape(1000,1))
X = scalarX.transform(X)
y = scalarY.transform(y.reshape(1000,1))
model = Sequential()
model.add(Dense(4, input_dim=2, activation='relu'))
model.add(Dense(4, activation='relu'))
model.add(Dense(1, activation='linear'))
model.compile(loss='mse', optimizer='sgd')
model.fit(X, y, epochs=50, verbose=1)
xpred, a = make_regression(n_samples=3, n_features=2, noise=0.1, random_state=1)
xpred = scalarX.transform(xpred)
ypred = model.predict(xpred)
for i in range(len(xpred)):
print("X=%s, Predicted=%s" % (xpred[i], ypred[i]))
This code is used to make a regression prediction using Keras. It starts by creating a dataset of 1000 samples with 2 features and some noise. The data is then scaled using the MinMaxScaler() function from sklearn. Next, a Sequential model is created with two hidden layers of 4 neurons each and an output layer of 1 neuron. The model is then compiled with the mean squared error (mse) loss function and the stochastic gradient descent (sgd) optimizer.
Finally, the model is fit to the data for 50 epochs and verbose output is enabled. The code then creates a new dataset of 3 samples with 2 features and some noise, which is then scaled using the same scalarX object as before. The model's predict() method is used to make predictions on this new dataset, which are then printed out along with their corresponding inputs.